Templates
author : | Florian Desbois, Jean Couteau |
---|---|
contact : | eugene-devel@list.nuiton.org or eugene-users@list.nuiton.org |
revision : | $Revision$ |
date : | $Date$ |
Like explained in the previous chapter (Generation process ), there are two ways to generate files :
- Use a Generator (template written in Java using Nuiton-processor)
- Use a Transformer (model transformation written in Java)
EUGene consider Generators and Transformers like generation templates with in common an input model. The different is the output, the Generator will write files whereas Transformer will load another model of the same type or not.
EUGene manipulate mainly the ObjectModel , so there is an Abstract for this kind of model manipulation : ObjectModelGenerator and ObjectModelTransformer.
Eugene implements also an easy solution for Java code generation. So a java generator and a transformer are available : JavaGenerator and ObjectModelTransformerToJava.
Here is the resulting hierarchy :
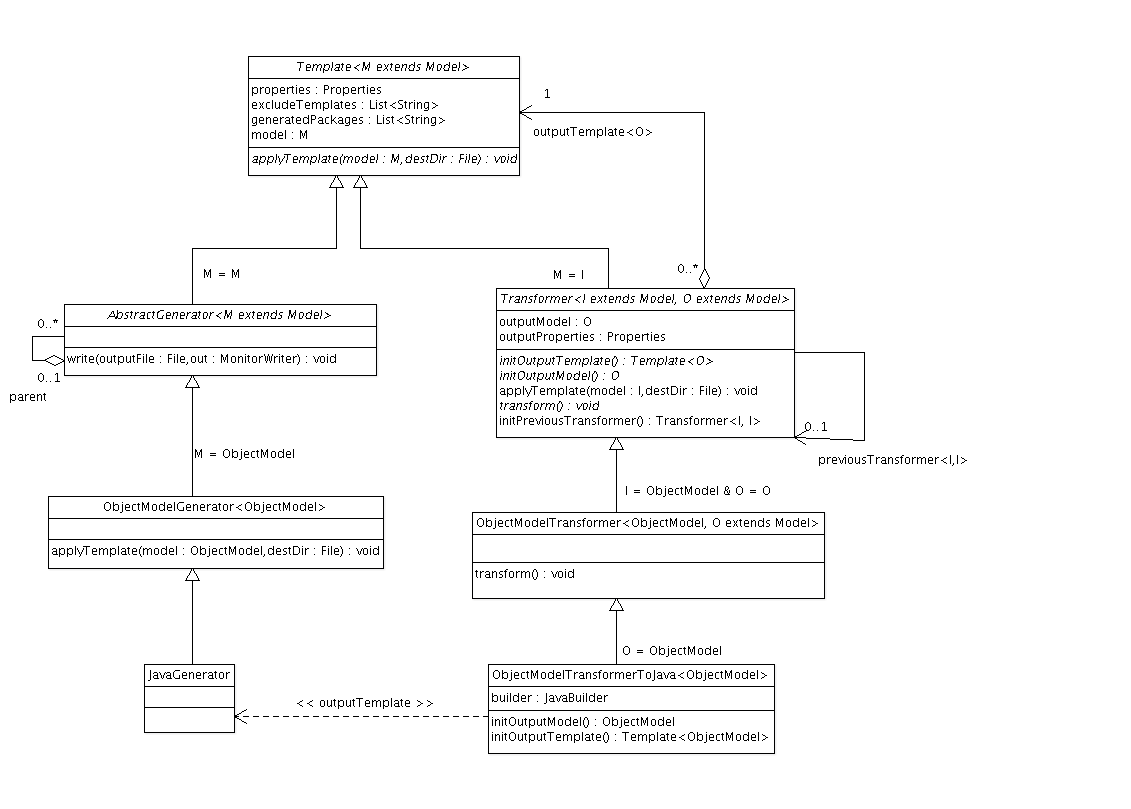
We will start by describing the writing syntax of a Generator using Nuiton-processor. Then we will describe Transformers' use to end with Java code generation.
Generator writing syntax
To create a Generator, you first have to create a new class that inherit from ObjectModelGenerator for generation from an ObjectModel's case.
TODO
Available Generators
- AbstractGenerator : input to define
- ObjectModelGenerator : ObjectModel input
- StateModelGenerator : StateModel input
- JavaGenerator : Java oriented ObjectModel (with extensions) input
- Note
- The maven plugin allows to know all the available transformers.
Model transformation implementation
As for Generators, Transformers work by inheritance. Everything depends on the wanted model types in input and output. For an ObjectModel input, you have to inherit from ObjectModelTransformer.
The transformer steps are :
- output initialisation
- transformer call before the actual transformation (useful for some chained transformations)
- input model transformation
- call the output template with the transformed model as input.
Transformer's input
Transformer's input is a Model. Usually, it is an ObjectModel but it is also possible to use other kind of models (like StateModel for example).
Transformer's output
It is necessary to initialise the Transformer's output :
- Output model : of what kind the output model is ?
- Output template : What is the template that will manipulate the output model ? That can be a Generator or another Transformer.
ObjectModelTransformer
ObjectModelTransformer features a simple pattern to transform models elements. All the ObjectModel will be run through and for each element type (Classifier, Class, Interface, Enumeration) a method will do the transformation, the developer will have to overload thos data to ease the transformation
public void transformFromModel(ObjectModel model) { } public void transformFromInterface(ObjectModelInterface interfacez) { } public void transformFromClass(ObjectModelClass clazz) { } public void transformFromClassifier(ObjectModelClassifier clazz) { } public void transformFromEnumeration(ObjectModelEnumeration enumeration) { }
ObjectModelTransformer is abstract and need an inheritance to specify the kind of model of the output (for Java code generation, it is also ObjectModel).
ObjectModelBuilder
This tool allows to fill an ObjectModel from a Transformer, obviously in the case the Transformer's output model is an ObjectModel. This class allows to ease ObjectModel's writing, the interfaces of this model not having setters.
Example of the available methods :
- createClass : create an ObjectModelClass
- createInterface : create an ObjectModelInterface
- addOperation : add a method to an ObjectModelClassifier (ObjectModelClass or ObjectModelInterface)
- addAttribute : add an attribute to an ObjectModelClassifier
- addParameter : add a parameter to an ObjectModelOperation
- ...
Available Transformer
- Transformer : input and output to define
- ObjectModelTransformer : ObjectModel input, output to define
- ObjectModelTransformerToJava : ObjectModel input, ObjectModel output, JavaGenerator as output template.
- Note
- The maven plugin allows to know all the available transformers.
Java generator
All the elements of the generation chain are available for a Java code generation from an XMI data model (org.nuiton.eugen.java package) :
- Output files : xmi, objectmodel, zargo, zuml
- input Model : ObjectModel
- output Model : Java oriented ObjectModel (with extensions)
- abstract Transformer : ObjectModelTransformerToJava
- output Generator : JavaGenerator
For each kind of wanted generation, you just need to inherit from ObjectModelTransformerToJava and use the transformFrom methods to record the Java oriented ObjectModel that will be automatically generated via JavaGenerator. The principle is to interpret the specificities of the input UML model to transform them into Java according to the technical needs (technology, framework, environment, ...).
JavaBuilder
To simplify the Java oriented ObjectModel writing, JavaBuilder is used transparently because ObjectModelTransformerToJava offering all its methods directly (delegation). So, the transformation is made via the input model run and those methods to load the output model.
Methods examples :
- createClass : create an ObjectModelClass
- createInterface : create an ObjectModelInterface
- addImport : Add an import to an ObjectModelClassifier
- addAnnotation : Add an annotation to an ObjectModelElement
- setSuperClass : Add a superclass to an ObjectModelClassifier (extends) with automatic superclass type import added.
- addConstant : Add a constant (static final) to an ObjectModelClassifier
- addConstructor : Add a constructor to a class.
- ...
- Note
- The imports management is done automatically on the types manipulated (attribute, parameter, method return, interface, ... type). This management uses the ImportsManagerExtension extension that manage an ImportsManager per classifier.